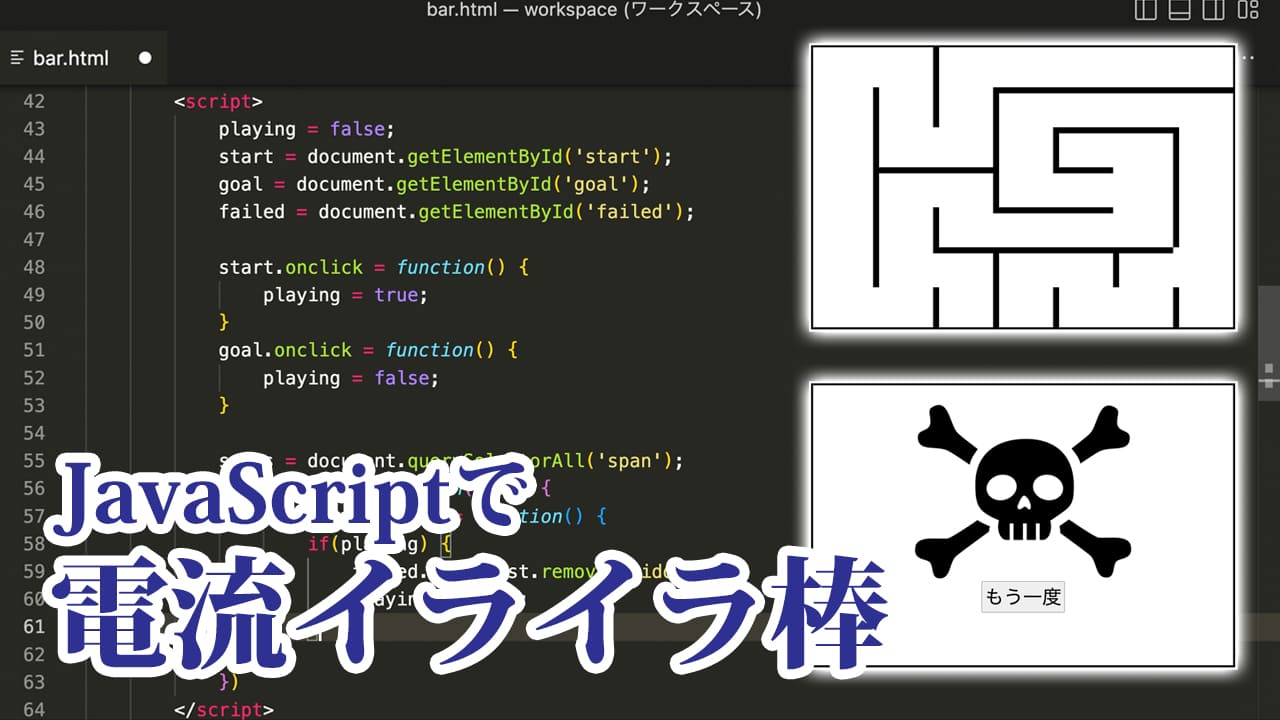
この記事を三行にまとめると
画面のデザインゲームの処理
壁を動かす
この記事は以下の動画の中に出てきたサンプルコードを載せたものです。コピペなどが必要なときに使ってください。
動かしたいspanタグにupやdonwのclassをつければ壁が動きます。
やっていることとは0.2秒ごとに壁の長さを100px→200px→100pxと変えているだけです。ただしこの場合は100pxと200pxの変化にしか対応してないので、他の長さの壁を動かす場合は別のCSSを用意する必要があります。
calcみたいなやつで「元の長さ+100px」的な書き方ができれば全部の長さに対応できて楽なんですけどね。そういうことはできないのかな……?
画面のHTML
<div id="box">
<div id="horizontal">
<span class="w4 t1 l3"></span>
<span class="w2 t2 l4"></span>
<span class="w2 t3 l1"></span>
<span class="w1 t3 l4"></span>
<span class="w2 t4 l3"></span>
<span class="w4 t5 l2"></span>
</div>
<div id="vertical">
<span class="h5 t1 l1"></span>
<span class="h2 l2"></span>
<span class="h1 t4 l2"></span>
<span class="h1 t6 l2"></span>
<span class="h3 t1 l3"></span>
<span class="h2 t5 l3"></span>
<span class="h1 t2 l4"></span>
<span class="h1 t6 l4"></span>
<span class="h1 t5 l5"></span>
<span class="h3 t2 l6"></span>
<span class="h1 t6 l6"></span>
</div>
<div id="failed" class="hidden">
<img id="image" src="failed.png" width="600" />
<div>
<button id="reset">もう一度</button>
</div>
</div>
<button id="start">S</button>
<button id="goal">G</button>
</div>
画面のCSS
//画面の大枠
#box {
position: relative;
margin: 0 auto;
width: 1050px;
height: 700px;
border: 5px solid;
}
//壁となる縦線と横線
span {
position: absolute;
display: inline-block;
}
#horizontal span { border-top: 15px solid }
#vertical span { border-left: 15px solid }
.w1 { width: 150px }
.w2 { width: 300px }
.w3 { width: 450px }
.w4 { width: 600px }
.w5 { width: 750px }
.h1 { height: 100px }
.h2 { height: 200px }
.h3 { height: 300px }
.h4 { height: 400px }
.h5 { height: 500px }
.t1 { top: 100px }
.t2 { top: 200px }
.t3 { top: 300px }
.t4 { top: 400px }
.t5 { top: 500px }
.t6 { top: 600px }
.l1 { left: 150px }
.l2 { left: 300px }
.l3 { left: 450px }
.l4 { left: 600px }
.l5 { left: 750px }
.l6 { left: 900px }
//アウト画面
#failed {
position: absolute;
padding: 40px;
text-align: center;
width: 100%;
height: 100%;
box-sizing: border-box;
background: #fff;
z-index: 100;
}
.hidden { display: none }
//ボタン
button { font-size: 48px }
#start, #goal {
position: absolute;
width: 80px;
height: 80px;
left: 495px;
border-radius: 50%;
}
#start { top: 10px }
#goal { bottom: 10px }
JavaScriptの処理
//ゲーム中かどうかの判定フラグ
playing = false;
//スタートボタン
start = document.getElementById('start');
start.onclick = function() {
playing = true;
}
//ゴールボタン
goal = document.getElementById('goal');
goal.onclick = function() {
playing = false;
}
//もう一度のボタン
reset = document.getElementById('reset');
reset.onclick = function() {
failed.classList.add('hidden');
}
//壁にぶつかったときにアウト画面を出す
failed = document.getElementById('failed');
spans = document.querySelectorAll('span');
spans.forEach(function(span) {
span.onmouseover = function() {
if(playing) {
failed.classList.remove('hidden');
playing = false;
}
}
});
壁を動かすアニメーション
.up {
animation:0.2s linear infinite up;
animation-direction: alternate;
}
.down {
animation:0.2s linear infinite down;
animation-direction: alternate;
}
@keyframes up {
0%{ height: 100px; }
100%{ height: 200px }
}
@keyframes down {
0%{ height: 100px; }
100%{ height: 200px }
}
動かしたいspanタグにupやdonwのclassをつければ壁が動きます。
やっていることとは0.2秒ごとに壁の長さを100px→200px→100pxと変えているだけです。ただしこの場合は100pxと200pxの変化にしか対応してないので、他の長さの壁を動かす場合は別のCSSを用意する必要があります。
calcみたいなやつで「元の長さ+100px」的な書き方ができれば全部の長さに対応できて楽なんですけどね。そういうことはできないのかな……?