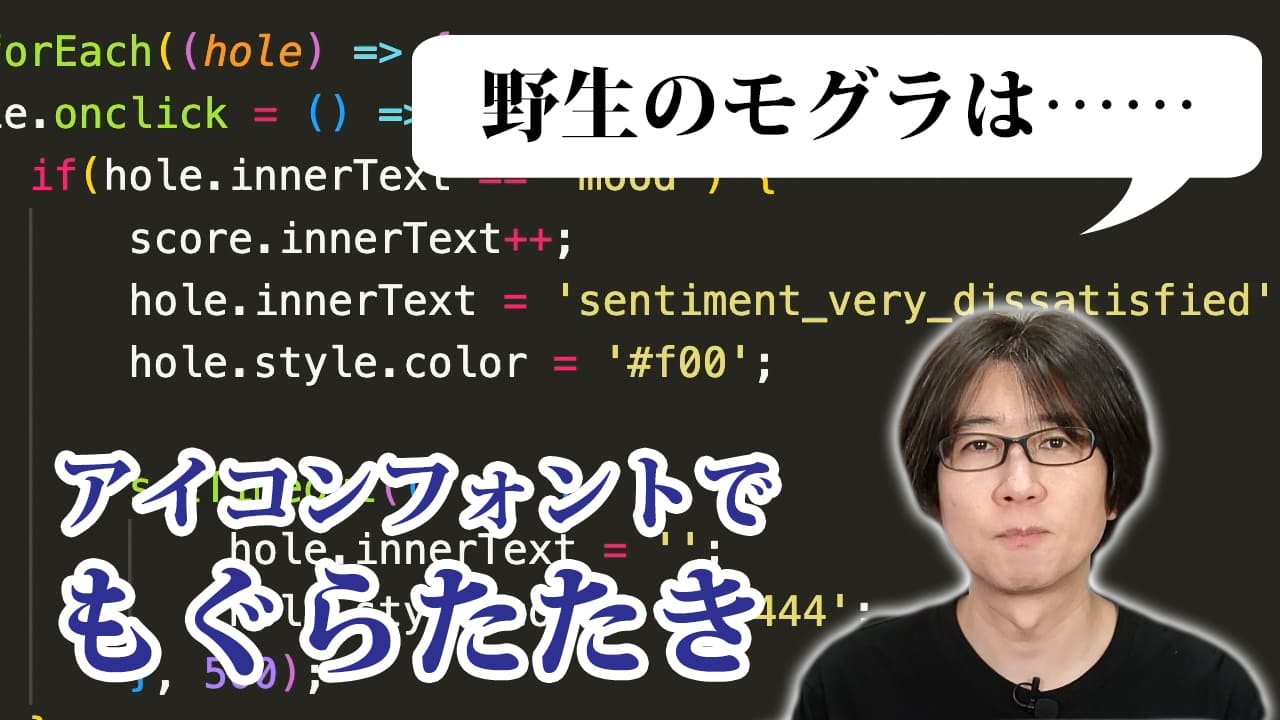
この記事は以下の動画の中に出てきたサンプルコードを載せたものです。コピペなどが必要なときに使ってください。
HTML
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="https://fonts.googleapis.com/css2?family=Material+Symbols+Outlined:opsz,wght,FILL,GRAD@24,400,0,0">
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="container">
<div class="hole"><span class="material-symbols-outlined">mood</span></div>
<div class="hole"><span class="material-symbols-outlined">mood</span></div>
<div class="hole"><span class="material-symbols-outlined">mood</span></div>
<div class="hole"><span class="material-symbols-outlined">mood</span></div>
<div class="hole"><span class="material-symbols-outlined">mood</span></div>
<div class="hole"><span class="material-symbols-outlined">mood</span></div>
<div class="hole"><span class="material-symbols-outlined">mood</span></div>
<div class="hole"><span class="material-symbols-outlined">mood</span></div>
<div class="hole"><span class="material-symbols-outlined">mood</span></div>
</div>
<div id="count">
<div id="score">0</div>
<div id="time">0</div>
<button id="start">START</button>
</div>
</body>
</html>
CSS
body { margin-top: 20px }
#container {
display: grid;
place-content: center;
grid-template-columns: repeat(3, 100px);
grid-auto-rows: 100px;
gap: 10px;
margin: 20px;
}
#count {
display: grid;
place-content: center;
grid-template-columns: repeat(3, 100px);
grid-template-rows: 60px;
gap: 10px;
margin-bottom: 20px;
}
#count div {
display: grid;
place-content: center;
border: solid 2px #444;
font-size: 24px;
}
.hole {
display: grid;
place-content: center;
cursor: pointer;
border: solid 2px #444;
}
.material-symbols-outlined {
font-size: 60px;
color: #444;
width: 60px;
height: 60px;
}
JavaScript
const score = document.getElementById('score');
const time = document.getElementById('time');
const start = document.getElementById('start');
const holes = document.querySelectorAll('.material-symbols-outlined');
// モグラの出現処理
appearance = (hole) => {
setTimeout(() => {
// 残り時間が0秒だったら処理終了
if(time.innerText == 0) {
hole.innerText = '';
return;
}
// アイコンのテキストを変える
hole.innerText = 'mood';
// 0.5〜3秒以内に自動で隠れる
setTimeout(() => {
if(hole.innerText == 'mood') {
hole.innerText = '';
// 次の出現処理
appearance(hole);
}
}, Math.floor(Math.random() * 2500) + 500);
}, Math.floor(Math.random() * 4000) + 1000);
}
// アイコンのクリックイベント
holes.forEach((hole) => {
hole.onclick = () => {
// 残り時間が0だったら処理終了
if(time.innerText == 0) {
return;
}
// アイコンがmoodだったらスコアを増やしてアイコンを変更
if(hole.innerText == 'mood') {
score.innerText++;
hole.innerText = 'sentiment_very_dissatisfied';
hole.style.color = '#f00';
// 0.5秒後にアイコンを消す
setTimeout(() => {
hole.innerText = '';
hole.style.color = '#444';
// 次の出現処理
appearance(hole);
}, 500);
}
}
});
// スタートボタン
start.onclick = () => {
score.innerText = 0;
time.innerText = 30;
// カウントダウンタイマー
let timerID = setInterval(() => {
if(time.innerText == 0) {
clearInterval(timerID);
} else {
time.innerText--;
}
}, 1000);
// アイコンを消して最初の出現処理をセット
holes.forEach((hole) => {
hole.innerText = '';
appearance(hole);
});
}