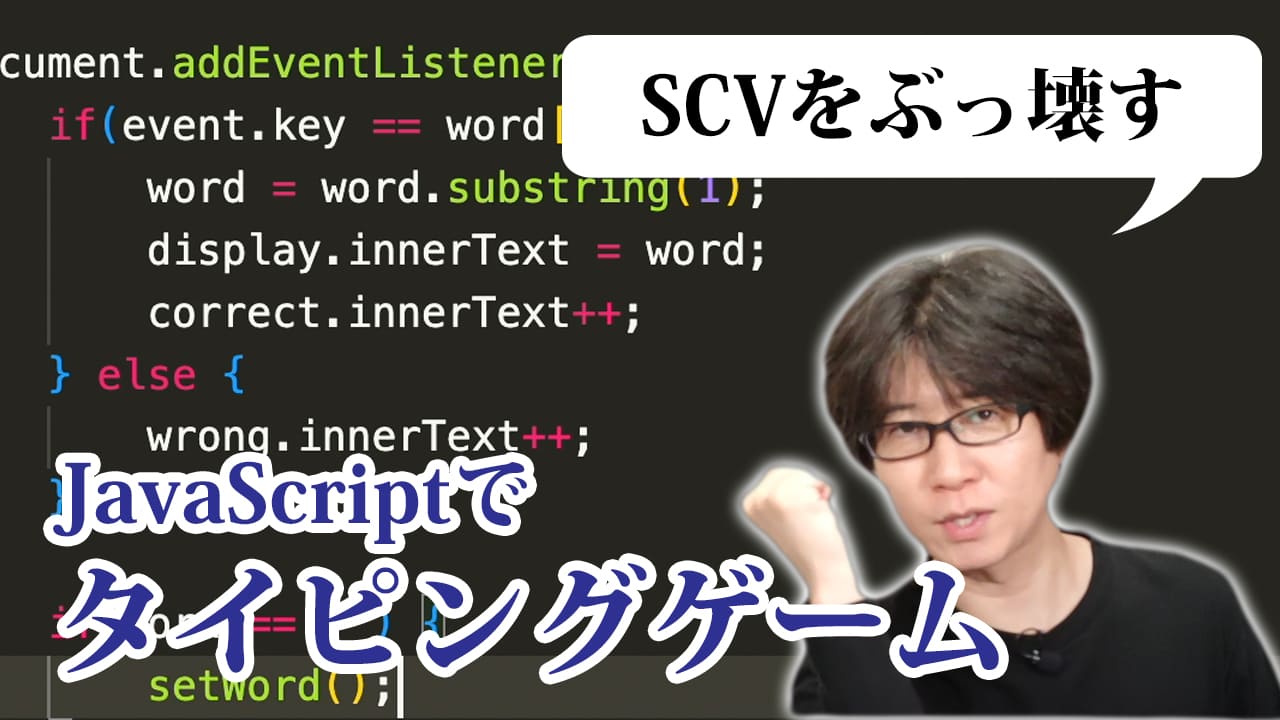
この記事を三行にまとめると
タイピングが速いことは無意味ではないみんなで年収10倍を目指しましょう
SCVをぶっ壊す
この記事は以下の動画の中に出てきたサンプルコードを載せたものです。コピペなどが必要なときに使ってください。
HTML
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="container">
<div id="display"></div>
<button type="button" id="start" autofocus="true">START</button>
</div>
<div id="count">
<div>正打:<span id="correct">0</span></div>
<div>ミス:<span id="wrong">0</span></div>
<div>残り時間:<span id="timer">0</span></div>
</div>
</body>
</html>
CSS
#container {
text-align: center;
margin-bottom: 20px;
}
#display {
font-size: 48px;
height: 80px;
}
#start {
font-size: 30px;
cursor: pointer;
}
#start:focus {
outline: none;
}
#count {
display: grid;
place-content: center;
grid-template-columns: repeat(3, 160px);
grid-template-rows: 60px;
}
#count div {
text-align: center;
font-size: 24px;
}
JavaScript
// タイピング用の単語
const words = [
'forEach', 'map', 'filter', 'if', 'switch', 'return', 'pop',
'continue', 'break', 'length', 'function', 'click', 'includes',
'querySelector', 'innerHTML', 'append', 'document', 'window',
'replace', 'split', 'const', 'let', 'setTimeout', 'setInterval',
'console', 'alert', 'true', 'false', 'undefined', 'push',
]
const display = document.querySelector('#display');
const start = document.querySelector('#start');
const correct = document.querySelector('#correct');
const wrong = document.querySelector('#wrong');
const timer = document.querySelector('#timer');
let word = '', end = true, index = 0;
// 単語をランダムに画面に出力する
setWord = () => {
n = Math.floor(Math.random() * words.length);
word = words[n];
display.innerText = word;
}
// スタートボタンを押したときの処理
start.onclick = () => {
end = false;
correct.innerText = wrong.innerText = 0;
// タイマー
timer.innerText = 10;
timerID = setInterval(() => {
timer.innerText--;
if(timer.innerText == 0) {
clearInterval(timerID);
end = true;
}
}, 1000);
setWord();
}
// キーを押したときの処理
document.addEventListener('keydown', (event) => {
// シフトキーは対象外とする
if(event.key == 'Shift' || end) {
return;
}
// 正解のときは文字を削除していく
if(event.key == word[0]) {
word = word.substring(1);
display.innerText = word;
correct.innerText++;
} else {
wrong.innerText++;
}
// 次の単語を出力する
if(word == '') {
setWord();
}
})