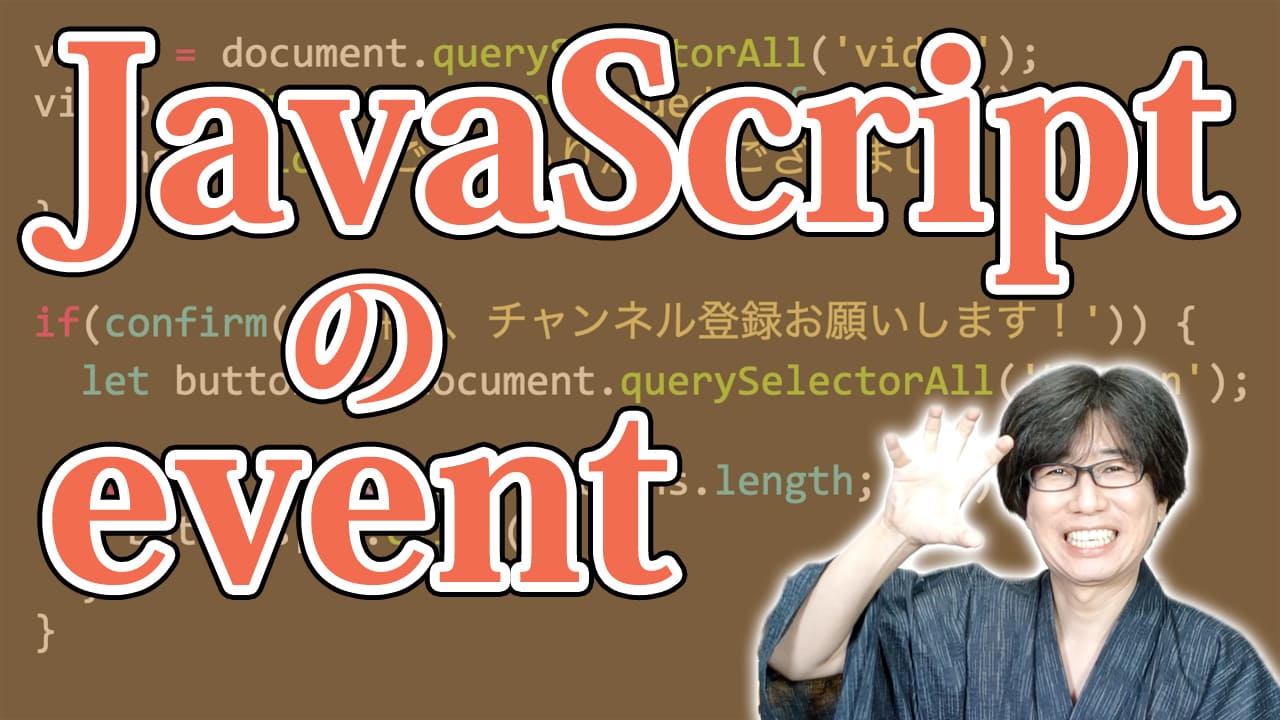
この記事を三行にまとめると
イベントの書き方イベントいろいろ
XMLHttpRequest
この記事は以下の動画の中に出てきたサンプルコードを載せたものです。コピペなどが必要なときに使ってください。
HTML要素の中にイベントを書く
<button onclick="alert('クリックしたよ!')">ボタン</button>
addEventListenerを使った書き方
<button id="button">ボタン</button>
<script>
button = document.getElementById('button');
button.addEventListener('click', function(){
alert('クリックしたよ!');
});
</script>
イベントハンドラに代入する書き方
<button id="button">ボタン</button>
<script>
button = document.getElementById('button');
button.onlick = function(){
alert('クリックしたよ!');
}
</script>
clickイベント
<input type="ckeckbox" id="checkbox" />
<script>
checkbox = document.getElementById('checkbox');
checkbox.onclick = function() {
if(this.checked) {
alert('チェックがついたよ!');
} else {
alert('チェックがはずれたよ!');
}
}
</script>
changeイベント
<select id="select">
<option value="東京">東京</option>
<option value="大阪">大阪</option>
<option value="福岡">福岡</option>
</select>
<script>
select = document.getElementById('select');
select.onchange = function() {
alert(this.value);
}
</script>
mouseoverとmouseoutイベント
<button id="button">ボタン</button>
<script>
button = document.getElementById('button');
button.onmouseover = function() {
this.style.backgroundColor = 'red';
}
button.onmouseout = function() {
this.style.backgroundColor = 'buttonface';
}
</script>
inputイベント
入力中の文字数:<span id="count">0</span>
<input type="text" id="text" />
<script>
text = document.getElementById('text');
count = document.getElementById('count');
text.oninput = function() {
count.innerHTML = this.value.length;
}
</script>
playとpauseイベント
<video id="video" controls>
<source src="movie.mov">
</video>
<script>
video = document.getElementById('video');
video.onplay = function() {
alert('動画を再生します');
}
video.onpause = function() {
alert('動画を一時停止します');
}
</script>
timeupdateイベント
<video id="video" controls>
<source src="movie.mov">
</video>
<script>
video = document.getElementById('video');
video.ontimeupdate = function() {
console.log(this.currentTime);
localStorage.setItem('time', this.currentTime);
}
</script>
loadイベント
window.onload = function() {
alert('ページの読み込みが完了しました!');
}
iframe = document.getElementById('iframe');
iframe.onload = function() {
alert('iframeの読み込みが完了しました!');
}
beforeunloadイベント
window.onbeforeunload = function(event) {
event.preventDefault();
event.returnValue = 'page change';
}
おまけ
XMLHttpRequestで新しいページを読み込む方法です。この場合、新しいページにあるloadイベントは発生しません。xhr = new XMLHttpRequest();
xhr.open('GET', '新しいページのURL');
xhr.send();
xhr.onreadystatechange = function() {
if(xhr.status == 200) {
document.body.innerHTML = xhr.responseText;
}
}