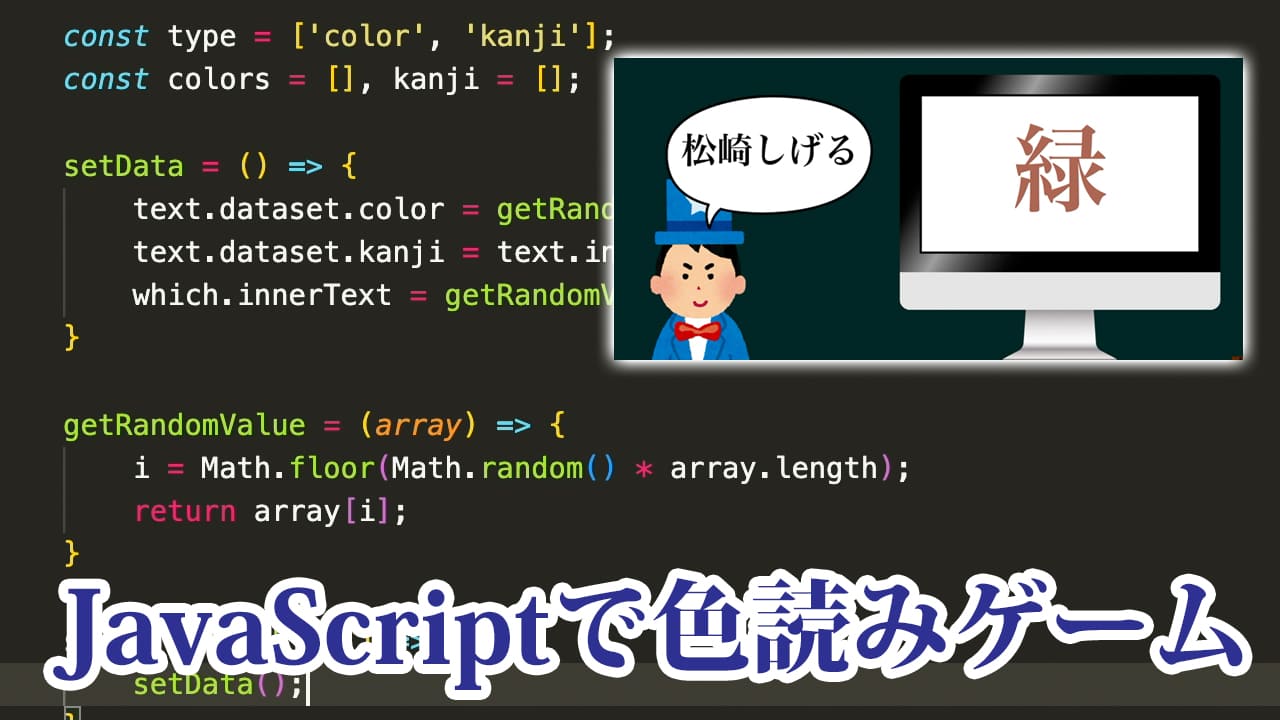
この記事を三行にまとめると
色つきの漢字をセットするdata属性を使って正解判定
ランダムだと意外と難しい
この記事は以下の動画の中に出てきたサンプルコードを載せたものです。コピペなどが必要なときに使ってください。
HTML
<div id="text" data-color="" data-kanji=""></div>
<div id="which"></div>
<div id="buttons">
<button data-color="red" data-kanji="赤"></button>
<button data-color="green" data-kanji="緑"></button>
<button data-color="blue" data-kanji="青"></button>
<button data-color="purple" data-kanji="紫"></button>
<button data-color="black" data-kanji="黒"></button>
</div>
<div id="count">
<div>正解数:<span id="correct">0</span></div>
<div>残り時間:<span id="timer">0</span></div>
</div>
<button id="start">START</button>
CSS
body {
width: 360px;
margin: 0 auto;
}
[data-color="red"] { --color: #f00 }
[data-color="green"] { --color: #090 }
[data-color="blue"] { --color: #00f }
[data-color="purple"] { --color: #f0f }
[data-color="black"] { --color: #000 }
#text {
color: var(--color);
text-align: center;
font-size: 96px;
height: 140px;
}
#which {
text-align: center;
font-size: 24px;
height: 40px;
}
#buttons {
display: flex;
justify-content: center;
gap: 10px;
button {
background-color: var(--color);
width: 64px;
height: 64px;
cursor: pointer;
border: none;
border-radius: 4px;
position: relative;
&:active {
top: 2px;
}
}
}
#count {
display: flex;
justify-content: space-between;
font-size: 24px;
margin: 20px 0;
}
#start {
width: 360px;
height: 40px;
cursor: pointer;
}
JavaScript
const text = document.getElementById('text');
const which = document.getElementById('which');
const start = document.getElementById('start');
const correct = document.getElementById('correct');
const timer = document.getElementById('timer');
const buttons = document.querySelectorAll('#buttons button');
const type = ['color', 'kanji'];
const colors = [], kanji = [];
// 漢字と答える種類を表示する
setData = () => {
text.dataset.color = getRandomValue(colors);
text.dataset.kanji = text.innerText = getRandomValue(kanji);
which.innerText = getRandomValue(type);
}
// 配列からランダムに値を一つ取得
getRandomValue = (array) => {
i = Math.floor(Math.random() * array.length);
return array[i];
}
// スタートボタン
start.onclick = () => {
correct.innerText = 0;
timer.innerText = 10;
setData();
// カウントダウンタイマー
timerID = setInterval(() => {
timer.innerText--;
if(timer.innerText == 0) {
clearInterval(timerID);
}
}, 1000);
}
// 回答ボタン
buttons.forEach((button) => {
colors.push(button.dataset.color);
kanji.push(button.dataset.kanji);
button.onclick = () => {
if(timer.innerText == 0) {
return;
}
// 答える種類(colorかkanji)を取得
answer = which.innerText;
// ボタンと表示中のcolorかkanjiが一致していたら正解数を+1
if(button.dataset[answer] == text.dataset[answer]) {
correct.innerText++;
setData();
}
}
});