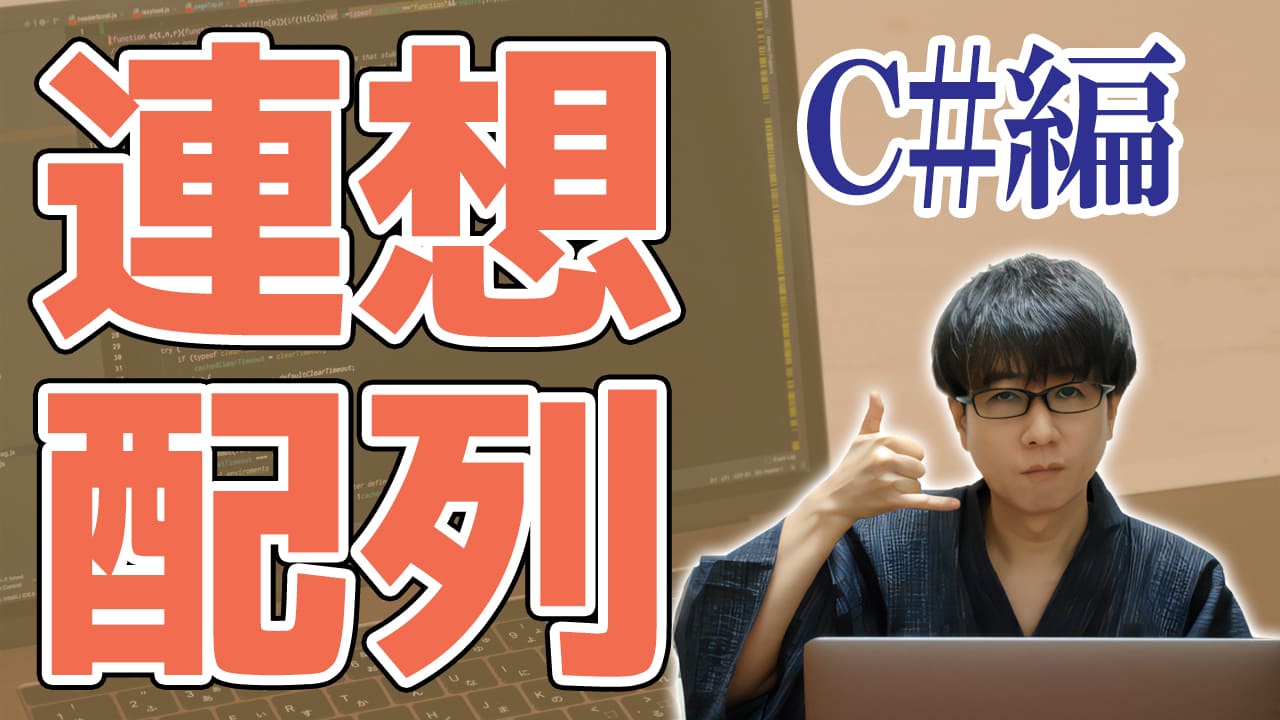
この記事を三行にまとめると
C#の連想配列要素の追加や削除
多重配列の書き方
この記事は以下の動画の中に出てきたサンプルコードを載せたものです。コピペなどが必要なときに使ってください。
基本的な書き方
using System.Collections.Generic;
Dictionary<string, int> points = new Dictionary<string, int>(){
{"国語", 75},
{"数学", 82},
{"英語", 100},
};
要素を取得
using System;
using System.Collections.Generic;
Dictionary<string, int> points = new Dictionary<string, int>(){
{"国語", 75},
{"数学", 82},
{"英語", 100},
};
Console.Write(points["国語"]); // 75
Console.Write(points["数学"]); // 82
Console.Write(points["英語"]); // 100
要素を追加
using System.Collections.Generic;
Dictionary<string, int> points = new Dictionary<string, int>(){
{"国語", 75},
{"数学", 82},
{"英語", 100},
};
// Add関数で追加
points.Add("社会", 58);
// 角かっこで追加
points["理科"] = 80;
要素の削除
using System.Collections.Generic;
Dictionary<string, int> points = new Dictionary<string, int>(){
{"国語", 75},
{"数学", 82},
{"英語", 100},
};
points.Remove("国語");
キーや値の存在確認
using System.Collections.Generic;
Dictionary<string, int> points = new Dictionary<string, int>(){
{"国語", 75},
{"数学", 82},
{"英語", 100},
};
// キーの存在確認
points.ContainsKey("国語"); // True
points.ContainsKey("社会"); // False
// 値の存在確認
points.ContainsValue("100"); // True
points.ContainsValue("50"); // False
Dictionaryの多重配列
using System.Collections.Generic;
Dictionary<string, Dictionary<string, int>> points = new Dictionary<string, Dictionary<string, int>>(){
{"太郎", new Dictionary<string, int>(){{"国語", 75}}},
{"花子", new Dictionary<string, int>(){{"国語", 90}}},
};
多重配列の要素を取得
using System;
using System.Collections.Generic;
Dictionary<string, Dictionary<string, int>> points = new Dictionary<string, Dictionary<string, int>>(){
{"太郎", new Dictionary<string, int>(){{"国語", 75}}},
{"花子", new Dictionary<string, int>(){{"国語", 90}}},
};
Console.Write(points["太郎"]["国語"]) // 75
Console.Write(points["花子"]["国語"]) // 90
多重配列に要素を追加
using System;
using System.Collections.Generic;
Dictionary<string, Dictionary<string, int>> points = new Dictionary<string, Dictionary<string, int>>(){
{"太郎", new Dictionary<string, int>(){{"国語", 75}}},
{"花子", new Dictionary<string, int>(){{"国語", 90}}},
};
points["太郎"]["数学"] = 80;
points["花子"].Add("数学", 70);
多重配列から要素を削除
using System;
using System.Collections.Generic;
Dictionary<string, Dictionary<string, int>> points = new Dictionary<string, Dictionary<string, int>>(){
{"太郎", new Dictionary<string, int>(){{"国語", 75}}},
{"花子", new Dictionary<string, int>(){{"国語", 90}}},
};
points["太郎"].Remove("国語");
多重配列のキーや値の存在確認
using System;
using System.Collections.Generic;
Dictionary<string, Dictionary<string, int>> points = new Dictionary<string, Dictionary<string, int>>(){
{"太郎", new Dictionary<string, int>(){{"国語", 75}}},
{"花子", new Dictionary<string, int>(){{"国語", 90}}},
};
points["太郎"].ContainsKey("国語");
points["花子"].ContainsValue(90);